Presenting The Python Book
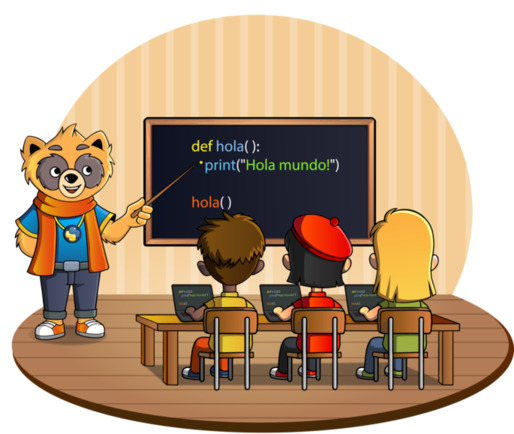
If you have arrived here, it is because you want to learn Python. With The Python Book, you will learn everything you need to incorporate this popular language into your daily life. We will cover the following topics:
- ๐จ The tools that Python offers: types, loops, conditionals, functions, and classes.
- ๐ How to use these tools effectively to solve problems.
- โ ๏ธ How to handle errors and exceptions when things go wrong.
- ๐งช How to write working code by creating tests.
- ๐ How to write clean, Pythonic, and easy-to-read code.
- ๐ค How to make informed decisions about what is best.
- ๐ 50 practical examples showcasing the potential of Python.
- โ 100 common mistakes in Python.
Letโs get started.
What is The Python Book?
The Python Book is a personal project that began during the COVID-19 pandemic in 2020 as a way to cope with the long days of isolation.
It started as a collection of quick personal notes in Spanish to review Python, but eventually evolved into this book. The Spanish edition was the first one published.
Basic programming skills are required to follow this book. You should have written some simple code before, know how to install Python, and run a program.
According to Paretoโs principle, 20% of the concepts are used 80% of the time. This book focuses on that 20% that will allow you to understand 80% of Python. It is akin to learning the most frequently used words in a language.
The book focuses on Python as a tool, not an end in itself. Programming is no longer just for programmers. It is for any professional who works with data, creates prototypes, conducts research, runs simulations, teaches, or automates tasks. The book aims to make Python accessible to everyone.
We emphasize practical aspects with real examples and set aside complex concepts that only advanced programmers use.
This is a living book. It is periodically revised and updated with your suggestions. If you have purchased it, you will receive new versions automatically via email.
It is a generalist book. It does not aim to cover and explain every concept in Python, but to provide the reader with the most commonly used tools and their practical applications.
What is not The Python Book?
This book is not meant to be a complicated manual filled with technical jargon and tedious to read.
It does not aim to cover every aspect of Python. Instead, it introduces the most commonly used Python concepts and packages that will enable you to accomplish a wide range of tasks.
It does not delve into theoretical aspects or advanced concepts that the average Python user might not be interested in. This book intentionally omits topics like metaprogramming, manipulation of the abstract syntax tree, or the use of the global interpreter lock, as 99% of people do not need to know these.
How to read The Python Book?
Repeat after me: You learn to program by programming. Simply consuming technical content like this book is ineffective if you do not put it into practice. Again, programming is learned by programming.
To get the most out of this book, it is recommended to have a computer at hand and work through all the examples. Instead of copying and pasting, write them down. Letter by letter. Line by line. This will help you understand it better.
Engage with the code presented in the book. Experiment with it. Modify it. Observe the outcomes. We recommend adopting a project-based approach to learning. Think of a small project you would like to create with Python and use it as a learning opportunity. Throughout the book, we will provide you with some ideas.
We suggest keeping Google handy to resolve any doubts. Websites like StackOverflow can be helpful. Do not forget about Large Language Models (LLMs) like ChatGPT. These tools can assist when you have questions or need suggestions. However, be cautious, as they can still make mistakes.
Almost all the code we present can be executed as is. In some cases, we include the output that the program produces, which makes it easier to follow.
You may not understand everything on the first read. That is normal. This book is a companion in your Python learning journey. Return to it whenever you need guidance.
Finally, congratulations on deciding to read The Python Book. Reading this book will undoubtedly place you ahead of 95% of people who write code by copying and pasting from Google, StackOverflow, and ChatGPT without understanding it and barely making it work.
Beyond Python
Programming extends beyond merely writing code. Just as knowing all the words of a language does not make you fluent, knowing Python syntax does not make you a proficient programmer.
Programming is an art where you use instructions to solve a problem. However, you must know how to use the right tools.
Although this book is about Python, its purpose is to solve problems through programming. Here are some tips on how to do this:
- ๐ง When a problem seems too complex, break it down into smaller, simpler subproblems. Divide and conquer. It is better to have many simple functions than a very complex one.
- โ๏ธ Do not reinvent the wheel. No matter how unique your problem is, someone else has likely tried to solve it before. Search the Internet.
- ๐๏ธ Be clear about the problem you are solving. A well-defined problem is half-solved. Never lose sight of it.
- โ๏ธ Be aware of the trade-offs. You cannot have everything. Sometimes more readable code is slower, or shorter code is unreadable. Make choices on a case-by-case basis. Every decision involves an opportunity cost, something you give up.
- ๐ช When you encounter a problem that seems unsolvable or you feel blocked, apply the rubber duck technique. Explain the problem to a rubber duck. Verbalizing it will help you.
- ๐งฉ Do not forget the difference between validating and verifying. The code may work, but if it does not solve your problem, it is useless. Technical profiles often focus too much on verifying and too little on validating.
- ๐ Software complexity can grow without limits. The best solution is the simplest one. You can kill flies with a cannon, but it is not the best approach. Perfection is achieved not when there is nothing more to add, but when there is nothing more to take away.
- โพ๏ธ Understand the problems that can arise when things go wrong. No matter how unlikely, manage these cases. Sooner or later, they will appear. Your code should be prepared. Some call this the Murphyโs Law.
Python pros/cons
Python has been at the top of the list of the fastest-growing and most-used programming languages for several years, and for good reason.
It has democratized access to programming, much like Gutenbergโs printing press democratized access to books, and Kodakโs cameras democratized access to photography.
Programming is no longer exclusive to programmers. Python is a simple language designed for everyone, but it is not suitable for every task. Like everything in life, it has its pros and cons.
๐๐ผ Python is very easy to use. You do not need to know complicated programming concepts to use it.
๐๐ผ It allows for rapid iteration, quickly turning an idea into code. This makes it very useful for rapid prototyping.
๐๐ผ Python has a large community, and there are thousands of packages that allow you to do almost anything.
๐๐ผ It manages memory automatically. You do not have to worry about allocating or freeing memory as you would in languages like C.
๐๐ผ Python can act as a wrapper for other programming languages, allowing interaction with more efficient code such as C or Rust.
๐๐ผ Python is not the fastest language available. It is relatively slow, especially when compared to lower-level languages.
๐๐ผ It is not the safest language. Python uses dynamic typing, which means that functions do not have a specific type at compile time. It is not used for writing critical avionics software.
๐๐ผ Python does not detect errors during compilation. Technically, it does not even compile. Other languages have a compilation step that acts as a first filter, protecting against certain errors. Python lacks this protection, making it less safe.
Python packages
A Python package is code organized under a single namespace. It is a way of grouping related code, and best of all, you can publish it so others can use it.
Python has a package manager called pip
, which allows us to publish and download packages created by others. If you want to solve a problem, check there because someone has probably already released a package that addresses it.
There is a package for almost everything, which makes Python a very rich language. Some well-known packages include:
numpy
: Numerical and scientific calculations.requests
: HTTP request management.matplotlib
: Graph and visualization representation.tensorflow
: Machine learning and artificial intelligence.flask
: Easy creation of web applications and APIs.biopython
: Bioinformatics and DNA manipulation tools.astropy
: Algorithms for astronomy.moviepy
: Video editing.quarto
: Web dashboard creation.
You can install any of them using pip
.
pip install numpy
Another advantage of pip
is that anyone can publish a package. If you have something to share with the world, you are welcome to do so. You will help others with your work.
However, remember that since anyone can publish packages, the quality can vary greatly. If you use an external package, check how many downloads or stars it has, investigate if others are using it, or look for anything unusual. Some packages may contain malicious code that can be very dangerous depending on your application. Be careful.
Pythonic code
In Python, there is the term Pythonic. Your code may or may not be Pythonic. Naturally, we want it to be.
Writing Pythonic code means following the languageโs principles and conventions. This usually results in code that is simple, readable, and takes full advantage of Pythonโs syntax.
This is similar to idiomatic expressions in a language. In Spanish, you say โpasarlo bienโ and in English, you would be understood if you said โto pass it well,โ but it is better to say โto have a good time.โ
Let us look at a couple of code examples. Both codes do the same thing and are perfectly valid, but one represents a Pythonic way of doing things, and the other does not.
โ This way of iterating a list with its index is not Pythonic.
# Not Pythonic
index = 0
for value in list:
print(index, value)
index += 1
โ This form is Pythonic.
# Pythonic
for index, value in enumerate(list):
print(index, value)
โ This way of checking if a number is even is not Pythonic.
# Not Pythonic
def is_even(number):
if number % 2 == 0:
return True
else:
return False
โ This form is Pythonic.
# Pythonic
def is_even(number):
return number % 2 == 0
We will explore this in detail later. The key takeaway is that while it is important to make things work, it is also beneficial to strive for Pythonic code. It makes it easier for others to read and simplifies the code. Remember that code is more often read than written.
Python, ChatGPT, and LLMs
In recent years, there has been a boom in Artificial Intelligence, ChatGPT, and LLMs (Large Language Models) in general.
These tools can do a little bit of everything, but in the context of programming, they can write code relatively well. Let us look at an example with ChatGPT.
โ We asked the following.
# I have a list l = [6, 4, 7, 81, 0].
# Write in Python a function that sorts it.
๐ค ChatGPT responds with the following code.
def sort_list(lst):
return sorted(lst)
l = [6, 4, 7, 81, 0]
sorted_list = sort_list(l)
print("Sorted list:", sorted_list)
# Sorted list: [0, 4, 6, 7, 81]
โ We tried another example. We asked.
# Write a function in Python to count
# how many vowels are in a text string.
๐ค It responds with.
def count_vowels(string):
vowels = "aeiou"
return sum(1 for char in string.lower() if char in vowels)
print(count_vowels("Hello World"))
# 3
In both cases, it provides the correct answer. These are simple examples, and a quick Google search would have yielded the same result, but it certainly saves time browsing through several web pages full of text looking for the answer.
These tools work relatively well, especially for simple questions. However, they become complicated when dealing with external packages that are not widely used, when context about a large code repository is needed, or with advanced concepts.
Even so, they help someone who does not know Python to express what they want in natural language and get a code in return. And that often works.
At this point, you may be thinking, โLearning Python is unnecessary; ChatGPT does it all for me. You do not need to know how to program anymore.โ
We do not believe this is true. Artificial Intelligences still have hallucinations, meaning they make up things that do not make sense. They work well with simple examples, but when things get complicated, they start to fail. Finally, someone with a good Python background can write better prompts, so knowing Python matters.
A prompt is simply the input, the question you give ChatGPT. In this book, among other things, we provide criteria for not accepting any nonsense that ChatGPT might produce.
It is true that these tools are improving at an incredible speed, and it is possible that in a few years, they will radically change the way we program.
To anticipate this change, we recommend that you start testing some of the following tools:
- ChatGPT: Created by OpenAI, the first chatbot. Use it to ask Python questions.
- Claude: Similar to ChatGPT.
- Cursor: A VSCode-based development environment that natively integrates any of the above. Use it to program in Python and โtalkโ with your code.
- DeepSeek: A Chinese LLM. The DeepThink (R1) feature is specially interesting as it gives you both the answer and the reasoning behind it.
An extra note about Cursor: ChatGPT and Claude are generic chatbots, but Cursor is a tool focused on software development and programming. It is a development environment or code editor with an integrated AI assistant. Some interesting features include:
- Real-time code prediction with interesting suggestions.
- The ability to provide context to your queries by including other files, documentation, or links.
That said, here are some tips for using Artificial Intelligence in programming:
- โ Include as much detail in your prompt as possible. Asking for code to process a few pieces of data is not the same as asking for millions. The more detail and context, the better.
- ๐ Ask for explanations of the code. It will help you understand things and avoid copying and pasting without understanding anything.
- โ ๏ธ Sometimes, the response may include code that does not work or compile. In that case, ask again, including the error you encountered.
- ๐ง Never copy and paste code from ChatGPT or similar tools without understanding it first.
As you can see, it is an excellent tool, but we must be critical of the answers it provides and not settle for the first response. Use it, but with caution.
First steps with Python
As with any programming language, our first step is to display the โHello Worldโ message on the screen. Create an example.py
file and add the following code.
print("Hello world")
# "Hello world"
You can execute it in different ways:
- With
python example.py
in your terminal. - With your favorite development environment, such as PyCharm, VS Code, or Cursor.
As you can see, we include a comment with the output that the code would produce if executed. We will show this throughout the book.
Now we will define some variables: one of type str
and the other of type int
.
name = "Alicia"
age = 20
print(f"{name} is {age} years old")
# Alicia is 20 years old
Now we create a list
, similar to an array in other languages. Lists allow you to store a set of several data points, in this case, integers.
ages = [18, 25, 32]
We perform some operations with our list
, such as calculating the average.
print(sum(ages)/len(ages))
# 25.0
We can also create functions.
def mean(ages):
return sum(ages)/len(ages)
And use them.
print(mean(ages))
# 25.0
Python has an interesting behavior in the following case. In other languages, this would give 0
.
print(2/4)
# 0.5
We can also add comments.
# We store the ages in a list
ages = [18, 25, 32]
We can iterate over lists.
for age in ages:
print(age)
To show you the humor of the creators of Python, there are some easter eggs. Run the following code.
import this
Or the following.
import antigravity
As you can see, Python accomplishes a lot with very little. The syntax is simple and closely resembles natural language. This sets it apart from other programming languages, which require years of study and are designed to be used by professionals.
Python gives anyone the ability to communicate with a machine to ask it to perform a specific task.